How to Build Secure and Scalable Authentication System with Node.js and MongoDB
Build a secure, scalable authentication system with Node.js, Express, MongoDB, and JWT. Learn best practices for protecting user data and scaling your app.
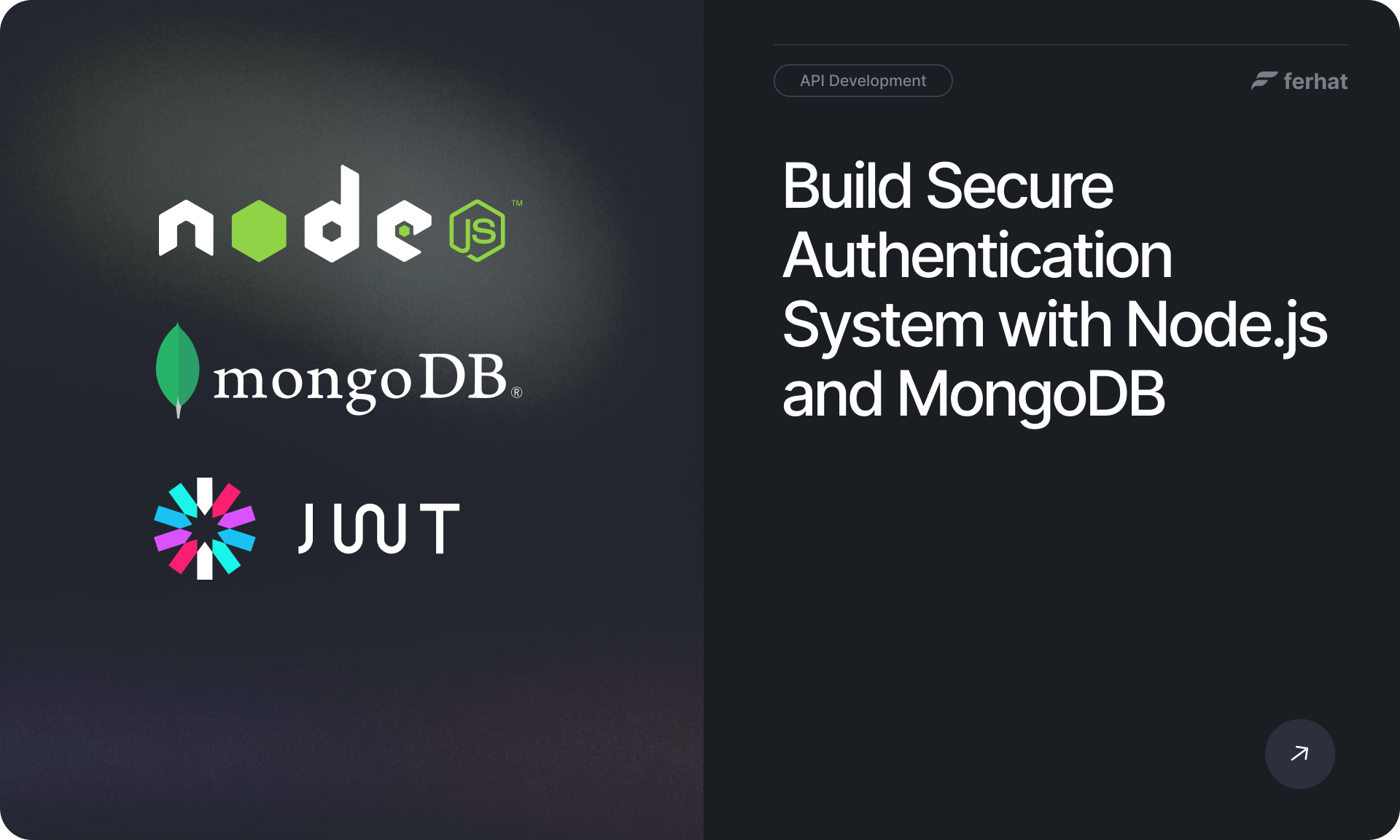
Understanding Authentication in Web Apps
Authentication is how we confirm a user’s identity to make sure they are who they say they are. It usually involves entering a username and password, which the system checks against its records. Today, many apps use more secure methods like Multi-factor Authentication or tokens like JSON Web Token. Good authentication protects data, keeps accounts safe and helps prevent unauthorized access.
In this guide, I’ll walk through building a secure and scalable authentication system using Node.js, Express.js, and MongoDB.
Step 1: Project Setup
Begin by creating a new Node.js project. Use TypeScript to ensure strong typing for better maintainability. Install the necessary dependencies:
2. Project Structure
Organize your project as follows:
Step 3: Configure Environment Variables
Create a .env
file in the root directory to store environment variables. Add the following variables:
Step 4: Setting Up the App Server
Create an app.ts
file in the src
directory to set up the Express app:
Step 5: Runnip App and Database connection
Create a server.ts
file in the src
directory to connect to the MongoDB database and start the Express server:
Step 6: Setting Up Models
Define a Mongoose schema for the User
model with fields like email
, name
, and password
. Make sure to hash passwords before saving them:
Step 7: Implementing Authentication
Create a routes/authRoutes.ts
file to define routes for user authentication:
Create a controllers/authController.ts
file to handle user authentication:
Step 8: Implementing User Routes
Create a routes/userRoutes.ts
file to define routes for user-related operations:
Step 9: Implementing User Controller
Create a controllers/userController.ts
file to handle user-related operations:
Step 10: Implementing Authorization
Create a middlewares/authMiddleware.ts
file to protect routes that require authentication:
Step Last: Enhancements and Best Practices
- Testing: Write unit and integration tests to ensure code quality.
- Rate Limiting: Protect your endpoints from abuse.
- HTTPS: Use HTTPS to encrypt data in transit.
- Monitoring: Implement logging and monitoring to detect and respond to issues.